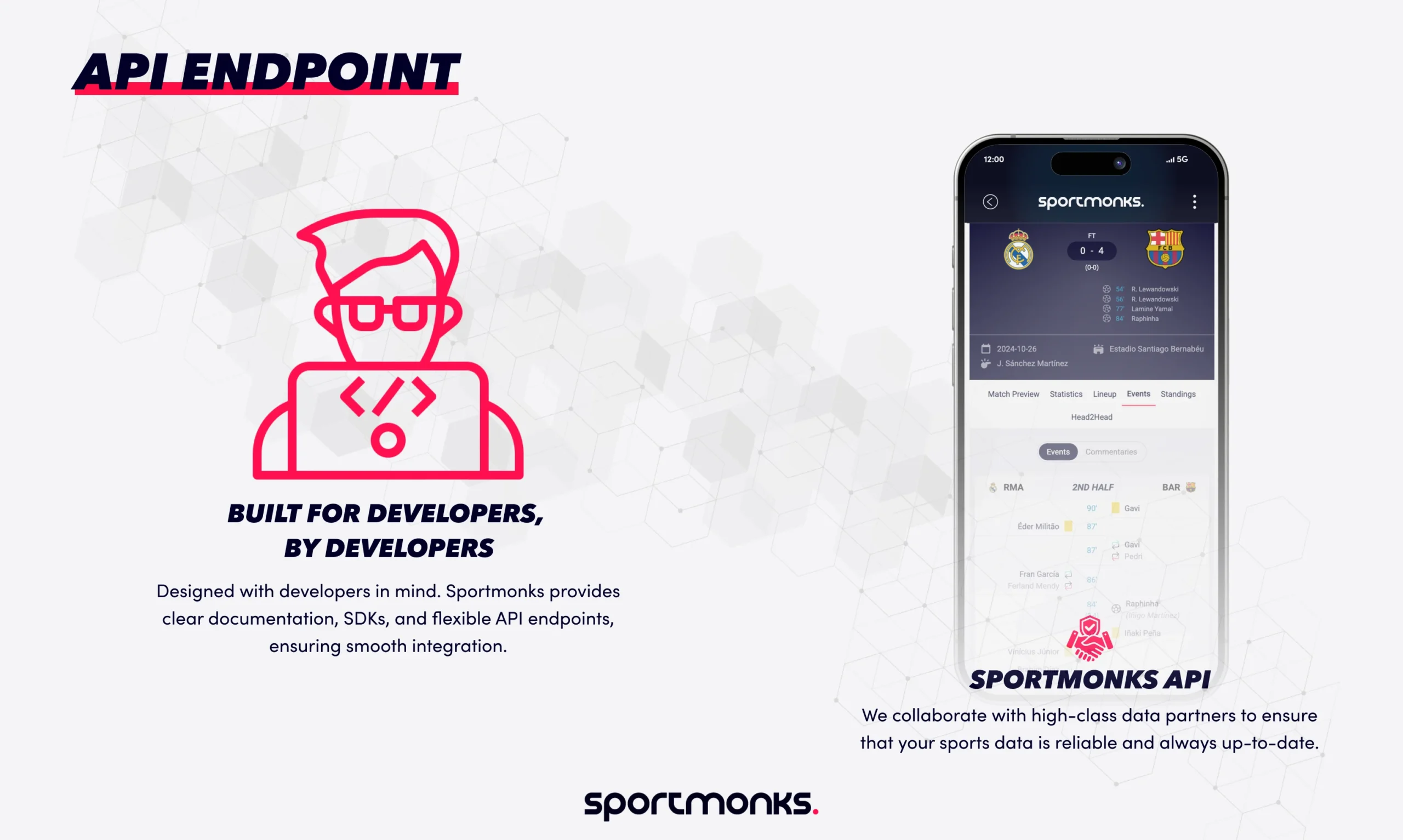
Contents
Key components of an API endpoint
Understanding the components of an API endpoint is crucial for effective API interaction—whether you’re fetching football stats, building integrations, or troubleshooting issues. Here’s a breakdown using insights and examples from the Sportmonks API.
URI (Uniform Resource Identifier)
A URI is the fundamental address for accessing resources on a server and serves as the core location of an endpoint. For the Sportmonks’ API, the URI follows RESTful conventions and includes three parts:
– Protocol: For example, HTTPS
– Domain: For example, api.sportmonks.com
– Path: For example, /v3/football/teams
Real-world examples
– The path /v3/football/leagues fetches league-related information.
– The path /v3/football/teams/{id} retrieves data for a specific team by its unique ID.
– For instance, accessing https://api.sportmonks.com/v3/football/teams/19 returns data associated with Arsenal FC, where 19 is the team’s unique ID.
HTTP method (e.g., GET, POST, PUT, DELETE)
HTTP methods such as GET, POST, PUT, and DELETE define the actions a client wants to perform on a resource, following the standards set by RFC 7231.
GET method
– Purpose: Retrieves data from the server.
– Characteristics:
Safe and repeatable; it doesn’t change server data.
Repeated requests return the same result.
Examples:
– GET /v3/football/leagues fetches a list of football leagues.
– GET /v3/football/fixtures/11935517 retrieves details for a match with fixture ID 11935517.
POST, PUT, and DELETE methods
– These methods are generally used to create, update, or delete resources.
– In the Sportmonks API, these actions are not available to the public, emphasizing its focus on data retrieval without modifying underlying data.
Request headers
Request headers are metadata sent with an HTTP request. They provide the server with important context and client requirements.
– Authentication: API endpoints like the Sportmonks API uses an API token for authentication, which can be provided in two ways:
Header-based authentication: Include your API token in the header using the Bearer scheme, for example:
Authorization: Bearer <your-api-token>
Query parameter authentication: Pass your API token as a query parameter in the URL, for example:
https://api.sportmonks.com/v3/football/fixtures?api_token=<your-api-token>
Accept header: The Accept header tells the server the desired response format. Sportmonks uses JSON by default, so you typically include:
Accept: application/json
While HTTP/1.1 headers are case-insensitive (can be written however you want), consistent capitalisation is recommended for readability and maintainability.
– Request body: The request body is the data payload sent to a server during operations that create or modify resources, typically used with HTTP methods like POST, PUT, and PATCH. Modern APIs mostly use JSON for this payload.
– Sportmonks’ API approach: Sportmonks is primarily a GET-heavy, read-only API. Instead of using a request body for passing parameters, it relies on query parameters within the URI. For example, appending ?include=players to the URI tells the server to include player data in the response.
Response headers
Response headers are metadata sent by the server along with its response. They provide important details about the returned data.
– Content-type: This header indicates the format of the response body. In the Sportmonks API, it typically shows as: Content-Type: application/json, which means the data is returned in JSON format.
– Rate-limit-remaining: This header informs you of how many requests you can still make within your current rate limit. For example, Sportmonks returns a rate_limit object containing the remaining requests that can be made, how long it takes to reset and the request entity (The data you requested for), so rate-limit.remaining: 2999 tells you that 2999 requests remain available.
Response body
The response body constitutes the actual data returned by the server, and in the case of Sportmonks, this data is delivered in JSON format, providing detailed sports information. Typically, the response body includes a “data” object containing the requested resource, along with supplementary metadata such as pagination details.
For example, a GET /v3/football/teams/19?include=players request returns information about Arsenal, including its team details and player roster:
{ "data": { "id": 19, "name": "Arsenal", "players": [{ "id": 123, "name": "Bukayo Saka" }, ...] }, "subscription": {...} }
In addition to the response body, HTTP status codes are returned, providing numeric indicators of the request’s outcome, adhering to established HTTP standards:
– 200 OK: Indicates a successful request, signifying that the requested data has been retrieved. For instance, a GET /v3/football/leagues request resulting in a 200 status code confirms successful retrieval of league data.
– 201 Created: Signifies that a resource has been successfully created. However, this status code is not applicable in the SportMonks API, as it primarily focuses on data retrieval.
– 400 bad request: Indicates that the request contained invalid parameters or syntax, leading to its failure.
– 404 not found: Signifies that the requested resource could not be found, typically due to an incorrect ID or endpoint. For example, a GET /v3/football/teams/999999 request, where 999999 is an invalid team ID, would result in a 404 status code.
– 500 internal server error: Indicates an issue on the server side. While rare, encountering this status code suggests retrying the request at a later time.
Sportmonks’ documentation provides a comprehensive list of common error codes, including 429, which signifies a rate limit breach.
Types of API endpoints
API endpoints enable data exchange and interaction between applications, and their design varies based on architectural styles and data needs. Here’s a breakdown of the primary types.
RESTful (Representational state transfer) endpoints
These endpoints follow REST principles and use HTTP methods (GET, POST, PUT, DELETE) to manage resources.
– Key traits:
Stateless: Each request is self-contained, carrying all the information needed.
Standard HTTP status codes: Uses codes like 200 OK or 404 Not Found to indicate outcomes.
Data format: Typically returns data in JSON, though XML or plain text are also possible.
– Use case: A GET request like https://api.sportmonks.com/v3/football/teams/19 fetches data for Arsenal FC in JSON format.
– Popularity: RESTful APIs are widely adopted due to their simplicity, efficient caching through HTTP, and scalability for web and mobile applications. RestAPI’s now have a 93.4% adoption rate, making it the most used API architecture among developers.
SOAP (Simple object access protocol) endpoints
SOAP endpoints utilise XML and a rigid, structured protocol for data exchange, emphasising strict adherence to standards.
– Key traits:
Standards-heavy: Uses WSDL (Web Services Description Language) to define service contracts.
Complex operations: Supports detailed operations with built-in security features like WS-Security.
Verbose: XML payloads lead to larger data transmissions compared to JSON.
Legacy dominance: Widely used in older enterprise systems, such as those in banking and telecommunications.
Use case: SOAP is ideal for internal, high-security applications that require guaranteed message delivery, such as payment processing systems. An example of a SOAP request example is;
<soap:Envelope> <soap:Body> <GetTeamInfo> <TeamID>19</TeamID> </GetTeamInfo> </soap:Body> </soap:Envelope>
– Trade-off: Although SOAP offers enhanced reliability, it is less flexible and more challenging to debug compared to REST.
GraphQL endpoints
GraphQL endpoints let clients precisely define the data they need and how it should be structured, offering a flexible alternative to traditional API designs.
– Key traits:
Single endpoint: A single endpoint (e.g., /graphql) handles all queries, streamlining API interactions.
Data efficiency: Clients avoid over-fetching or under-fetching data by specifying exactly what they need.
JSON and schema: Responses are returned in JSON format, and a schema defines the available data types and relationships.
Adoption growth: Popularised by GitHub’s adoption in 2017, GraphQL has become a standard for complex applications.
– Use case: Ideal for applications needing precise data retrieval, such as a football app fetching specific player statistics without extra data. An example of a GraphQL query example is;
query { team(id: 19) { name players { name } } }
And the response is;
{ "data": { "team": { "name": "Arsenal", "players": [ {"name": "Bukayo Saka"} ] } } }
– Strengths: GraphQL excels in efficiency and flexibility, allowing clients to dictate the shape of the response, minimising unnecessary data transfer and enhancing performance.
WebSocket endpoints
WebSocket endpoints enable real-time, two-way communication between clients and servers, allowing continuous data exchange.
– Key traits:
Persistent connection: Unlike the HTTP request-response model, WebSockets maintain an open connection for ongoing data transfer.
Low latency: They offer minimal delay, making them ideal for applications needing instant updates.
Protocols: WebSockets use ws:// for unsecured and wss:// for secured connections.
Adoption: With the rise of HTML5, WebSockets have become popular for real-time applications, powering over 6.3% of web applications (Georgia Institute of Technology).
– Use case: WebSockets are particularly well-suited for applications that require live updates, such as real-time sports scores, chat applications, and multiplayer online games. While the SportMonks API is REST-based and does not currently utilise WebSockets (It offers a livescore api endpoint for ongoing events), a hypothetical WebSocket endpoint, such as wss://api.sportmonks.com/football/fixtures, could be used to stream real-time match updates. A client subscribing to wss://api.sportmonks.com/live might receive an instant update in the following format: {“event”: “goal”, “team”: “Arsenal”}.
RPC (Remote procedure call) endpoints
RPC endpoints allow clients to invoke functions on a remote server as if they were local calls.
– Key traits:
Procedure-focused: The calls are function-oriented (e.g., getTeam(19)), unlike REST which is resource-based.
Data formats: Can utilise JSON (as in gRPC or JSON-RPC) or binary formats (like Protocol Buffers).
Performance: Often lightweight and fast; for example, gRPC—a modern RPC framework by Google—is 5-10 times faster than REST for microservices.
– Use case: Common in internal systems or microservices—e.g., a backend calling calculateStats(teamId). An example of a JSON-RPC call:
{"jsonrpc": "2.0", "method": "getTeam", "params": [19], "id": 1}
Response:
{"result": {"name": "Arsenal"}, "id": 1}.
– Why it’s niche: Simpler for devs familiar with function calls, but less web-friendly than REST.
Purpose of API endpoints
– Getting data: API endpoints allow applications to retrieve up-to-date data from external sources like databases or other services, eliminating the need to store information locally.
– Transforming data: They enable data manipulation—creating, updating, and deleting records in remote systems—so applications can modify data in real time.
– Integrating with services: Endpoints facilitate integration with third-party services such as payment gateways, social media, and mapping platforms, adding advanced functionality without extensive in-house development.
– Communicating with apps: They are essential for inter-application communication, allowing different systems to exchange data and trigger actions, which is key for distributed systems and microservices architectures.
– Automation: API endpoints help automate tasks and workflows like data processing, report generation, and system monitoring, boosting efficiency and reducing manual work.
– Building mobile apps: In mobile development, endpoints enable apps to access remote data and services, delivering rich, dynamic content to users.
– Web app development: They support the creation of dynamic web applications that update content without requiring a full page reload, enhancing the user experience.
– Microservice architecture: Endpoints act as the communication channel between microservices, ensuring efficient interaction among various components in a system.
Security considerations for API endpoints
Keeping API endpoints secure is vital to protect private information, stop unwanted access, and maintain user confidence. With strong security measures, you can build a reliable API system. Below are key steps to make this happen.
Authentication
Authentication checks who is trying to use the API before letting them in, acting as the first layer of security. One way is using API keys, which are special codes often included in the Authorisation header—like “Authorisation: Bearer abc123xyz”—or added to the web address, as seen in a Sportmonks’ example:
https://api.sportmonks.com/v3/football/livescores?api_token={{api_token}}
Another method is OAuth 2.0, which uses tokens to safely allow third-party access, such as “Login with Google.” There’s also JSON Web Tokens (JWT), which are coded tokens carrying user details for the server to check. For the best protection, require authentication for every endpoint—even the “public” ones—so you can track usage and prevent unauthorised entry.
Authorisation
Authorisation makes sure that users who are already checked by authentication can only access the parts of the API they’re allowed to use. It controls what resources or actions they can reach. One way to do this is with Role-Based Access Control (RBAC) or Attribute-Based Access Control (ABAC), which set detailed permissions based on a user’s role or traits. For example, someone on a basic plan might be able to use GET /v3/football/teams but would get a “403 Forbidden” error if they tried to access premium features like /v3/football/statistics. The best approach is to apply specific authorisation checks for each action, rather than depending only on authentication to decide access.
HTTPS encryption
HTTPS encryption keeps data safe while it travels between the user and the API by locking it with a secure code. This is important because, without HTTPS, private details like API keys could be stolen by someone watching the network. For example, all SportMonks API endpoints, such as https://api.sportmonks.com/v3/football/leagues, require HTTPS to protect the connection. The best way to handle this is to use modern security standards like TLS 1.2 or 1.3 and automatically switch any HTTP requests to HTTPS to ensure everything stays secure.
Input validation
Input validation stops harmful attacks, like SQL injection or XSS, by checking and cleaning up what users send to the API. This means making sure the data matches expected types, lengths, and formats, and removing risky symbols or code. For example, a request like GET /v3/football/teams/<script>alert(1)</script> should be blocked or fixed to prevent trouble. The best way to do this is to use trusted tools, such as validation libraries like Joi or express-validator, and never assume raw user input is safe.
Rate limiting
Rate limiting stops misuse and denial-of-service (DoS) attacks by setting a cap on how many requests a user can send to the API. This is done by limiting requests for each client—for example, allowing 1000 requests per day—based on their API key or IP address. In Sportmonks, you might see a header like rate-limit.remaining: 999, and if you go over the limit, you’ll get a “429” error. The best approach is to pair rate limiting with throttling to control traffic smoothly and keep the experience better for users.
API key management
API key management protects API keys to stop unauthorised access from happening. This involves keeping keys safe, such as storing them in secure places like environment variables or special vaults like AWS Secrets Manager. It’s also important to update keys regularly and cancel any that get exposed right away. For example, if a key like abc123xyz leaks, someone could take over a user’s request limit. The best way to lower this risk is to use short-lived API keys or OAuth tokens that don’t last long.
Logging and monitoring
Logging and monitoring keep track of what’s happening with the API and help spot anything strange or risky, like potential security breaches. This is done by recording details of requests, their results (like status codes), and any errors, so there’s a clear history to check. It also means watching for odd behaviour, such as sudden jumps in traffic or lots of errors. For example, you might log a normal request like GET /v3/football/teams/19 with a “200 OK” result, but also note unusual patterns, like a flood of “404” errors. The best way to manage this is to use special tools, such as Splunk or ELK Stack, and set up warnings for serious problems.
CORS (Cross-origin resource sharing)
CORS, or Cross-Origin Resource Sharing, decides which websites can use your API, stopping unapproved requests from other sources. This is managed by setting up the Access-Control-Allow-Origin header to name the trusted websites. For example, you might limit access to a specific site like https://myapp.com instead of allowing everyone with a wildcard (*). The best way to do this is to clearly list the permitted websites when your API is in use, especially in live settings.
Regular security audits
Regular security audits help find weak spots in the API before they can be taken advantage of. This involves running tests, like penetration tests and vulnerability scans, using tools such as OWASP ZAP, to check for problems. Any issues found should be fixed quickly. For example, an audit might show that an old version of TLS is being used or uncover other security gaps. The best approach is to follow the OWASP API Security Top 10 guidelines and use tools like npm audit to keep dependencies up to date.
Data sanitisation
Data sanitisation makes sure that API responses only show information that users are allowed to see. This is done by removing or hiding sensitive details, like email addresses or passwords, depending on what the user is permitted to access. For example, a request like GET /v3/football/players might return {“name”: “Saka”} without revealing private information. The best way to handle this is to filter the data on the server side, so you don’t depend on the user’s device to hide anything.
Best practices for designing and implementing API endpoints
Building API endpoints well means following clear rules to make them easy to use, secure, and reliable. Below are key practices to guide the process.
Use RESTful principles
RESTful principles help organise how the API works by linking HTTP methods—like GET, POST, PUT, and DELETE—to basic actions (create, read, update, delete) and using proper status codes, such as “200 OK” for success or “404 Not Found” for errors. For example, GET /v3/football/teams/19 fetches Arsenal’s details in a simple, RESTful way. The best approach is to use nouns for resource names, like /teams, and avoid verbs like /getTeams.
Design for simplicity and consistency
Keep API paths clear and logical, such as /leagues/{id}/teams, and stick to a steady naming style, like camelCase, snake_case, or kebab-case. For instance, endpoints like /v3/football/fixtures and /v3/football/teams follow a predictable pattern. The best way is to avoid complicated nesting, such as deep paths like /v1/football/leagues/teams/players/stats.
Version your APIs
Add version numbers to the API, either in the web address—like /v1/users—or in headers, such as Accept: application/vnd.example.v1+json. For example, https://api.sportmonks.com/v3/football/leagues uses “v3” to show it’s the third version. It’s wise to keep older versions running for 6–12 months after they’re phased out, so users can switch smoothly.
Document your APIs
Provide clear guides that explain endpoints, parameters, authentication, examples, and errors, using tools like Swagger. For instance, Sportmonks documents endpoints like GET /v3/football/teams/{id} with sample JSON responses. The best method is to add interactive “Try It” options so users can test the API easily.
Implement error handling
Send clear JSON responses with details like status, a message, and a code, matched with the right HTTP status code. For example, a faulty request to GET /v3/football/teams/xyz might return {“error”: “Invalid team ID”, “code”: 400}. The best practice is to use 400-series codes for user mistakes and 500-series for server issues, with helpful messages.
Optimise performance
Make responses faster by keeping data small, storing results in a cache, and compressing files with tools like Gzip. For example, GET /v3/football/fixtures?include=teams lets users choose extra data without overloading. It’s good to cache unchanging data, like team lists, for 5–15 minutes to speed things up.
Test thoroughly
Check the API with unit tests, integration tests, and full end-to-end tests to find problems early. For example, test GET /v3/football/leagues with both valid and invalid API keys. The best way is to use automated testing tools like Postman or Jest to save time.
Monitor and log
Record request details, responses, and errors, and watch for slow performance or rising error rates to spot issues. For instance, logs showing lots of GET /v3/football/teams/19 requests might mean optimisation is needed. The best tools for this are ones like Prometheus or Datadog, which can send alerts and insights.
Use standard data formats
Stick to JSON as the main format for responses because it’s consistent and easy to work with. For example, a response like {“data”: {“id”: 19, “name”: “Arsenal”}} follows this standard. It’s best to use JSON unless older systems need something different.
Consider pagination and filtering
Add options like page, limit, and filter to handle big sets of data efficiently. For example, GET /v3/football/fixtures?per_page=25&page=1 shows pagination in use. The best default is 20–50 items per page, letting users filter what they need.
Design for scalability
Prepare for more traffic by using load balancers, adding more servers, and keeping the design stateless. SportMonks uses load balancing to handle users worldwide. The best method is to keep REST stateless and use CDN caching for better performance as the API grows.
Follow security best practices
Keep endpoints safe with authentication, HTTPS encryption, input checks, and rate limiting. SportMonks, for example, uses HTTPS and rate limits, like rate-limit.remaining: 999. The best way is to follow the OWASP API Top 10 security guidelines to protect the API fully.
Tools and technologies for API endpoint development and management
Using the right tools and technologies makes building and managing API endpoints easier, faster, and more reliable. Below are the main options to consider.
Programming languages and frameworks
Programming languages and frameworks provide the base for creating APIs, offering structure and ready-made features to speed up coding. Popular choices include Python, where Flask is simple and lightweight, or Django with Django REST Framework offers more built-in tools. Node.js with Express.js is quick and straightforward, using JavaScript throughout. Java’s Spring Boot suits large, business-level projects, while Ruby on Rails is great for fast prototyping with its clear rules.
API gateways
API gateways handle incoming traffic, enforce security, and help the API grow with demand. Top tools include AWS API Gateway, which is serverless and pairs with Lambda, Azure API Management for big projects with detailed tracking, and Kong, an open-source option with many add-ons. They manage authentication, rate limiting, caching, and routing. For example, SportMonks likely uses a gateway to control requests like GET /v3/football/fixtures.
API documentation tools
Documentation tools make APIs easier to use by providing clear, interactive guides. Key options are Swagger/OpenAPI for creating detailed, testable specs, Postman for both testing and documenting, and Insomnia for a simple REST-focused choice. Sportmonks, for instance, has a documentation page and a Postman collection.
Version control systems
Version control systems track changes and help teams work together. The standard is Git for managing updates, paired with GitHub or GitLab for storing code, automating tasks, and team collaboration. In fact, about 93% of developers use Git, according to Stack Overflow 2023.
Testing tools
Testing tools check that the API works properly and find problems early. These include unit testing with tools like JUnit, pytest, or Mocha, integration testing to check connected parts, and end-to-end testing with Postman or ThunderClient. The best way is to write test scripts and run them every time the API is updated, using tools like Postman or Jest.
Containerisation and orchestration
Containerisation and orchestration ensure the API runs the same way everywhere and can handle more users. Docker packages the API into containers, while Kubernetes manages groups of containers. These tools make deployment steady and scaling simpler.
Cloud platforms
Cloud platforms provide strong foundations and services that grow with the API. The main ones are AWS, with options like Lambda and ECS, Azure, offering App Services and Functions, and Google Cloud, with Cloud Run and Firestore. It’s smart to use serverless options for handling sudden traffic spikes.
Monitoring and logging tools
Monitoring and logging tools track how the API performs and spot issues. Prometheus gathers metrics, Grafana shows them in dashboards, and ELK Stack handles logs. For example, watching delays on GET /v3/football/fixtures can reveal performance problems. It’s best to log 4xx and 5xx errors and set up alerts for them.
Security tools
Security tools protect APIs from risks and attacks. These include vulnerability scanners like OWASP ZAP or Burp Suite, authentication helpers like OAuth2 libraries or Keycloak, and penetration testing tools. Sportmonks’ use of HTTPS and API keys fits with tools like ZAP for testing. It’s also wise to scan for security issues every three months.
Databases
Databases store and manage the API’s data. Options include SQL databases like MySQL or PostgreSQL for structured data, and NoSQL ones like MongoDB or Redis for more flexible setups. For example, GET /v3/football/teams likely pulls from a relational database. Caching popular data in Redis can make things faster.