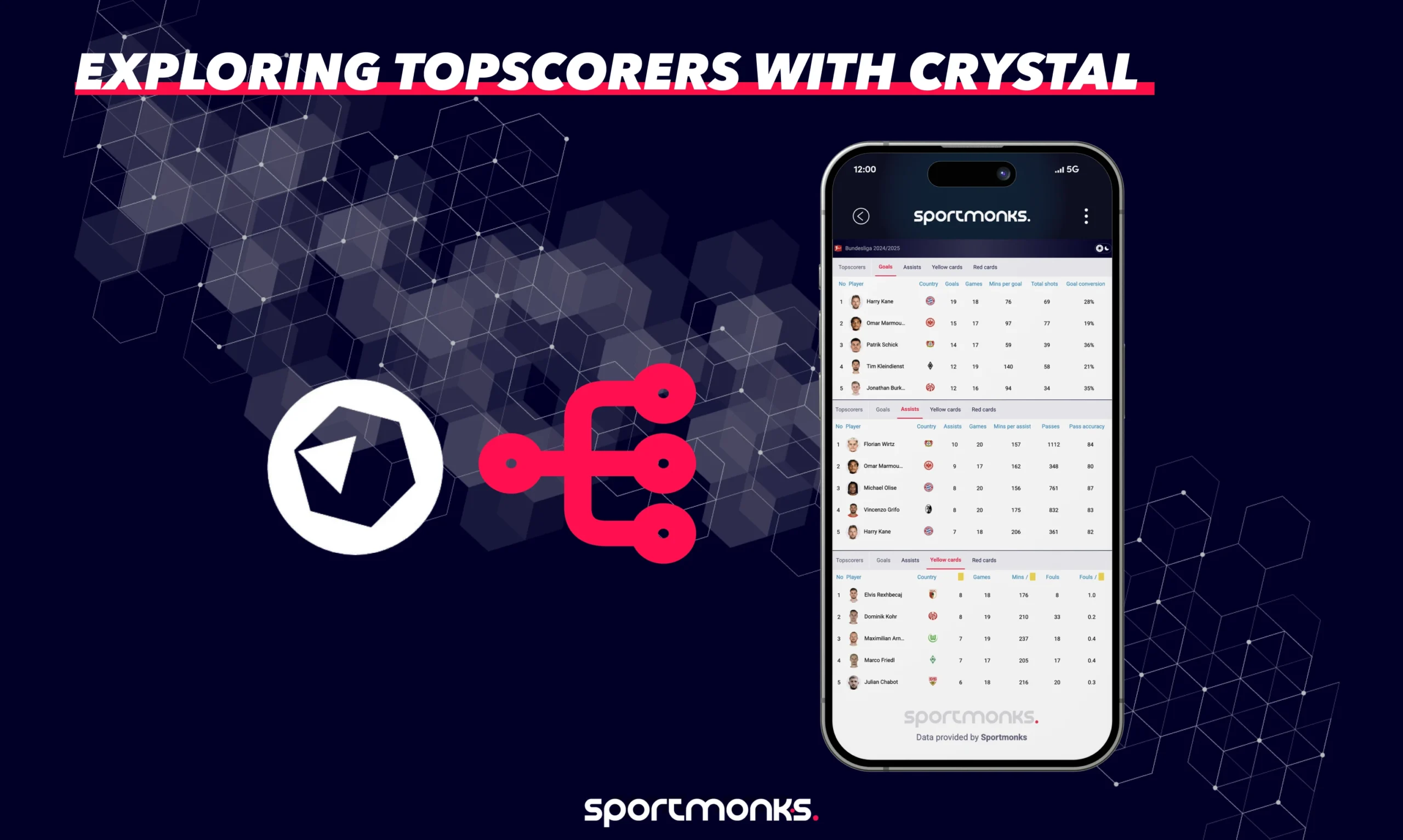
Contents
What is Crystal Programming Language?
Designed and developed by Ary Borenszweig, Juan Wajnerman, Brian Cardiff, and more than 400 contributors, Crystal, which is currently in active development, is a high-level general-purpose, object-orientated programming language.
The Crystal compiler was first written in Ruby but later rewritten in Crystal, leading to the first official version being released in June 2014.
Adoption – Who Uses Crystal?
While Crystal is not particularly popular in the programming world, it has been adopted in several fields such as biometrics, networking, cyber security, SaSS, and several gaming projects.
Chapter 1: Setting up your environment
To write and compile Crystal, we need to set up an environment to do so. Refer to the installation guide on the official documentation page for the method more suitable to your workstation.
Kindly be informed that Crystal is currently in active development and was released as free and open-source software under the Apache License version 2.0; hence certain features may be unavailable.
Assuming you’ve got yourself up and running after installing Crystal, you will need to obtain an API token.
1.1 Obtaining an API Token
Before we can begin to explore top scorers with Sportmonks Football API, we need to sign in to your account.
If you don’t have an account, you can take advantage of the 14-day free trial on any of our plans.
Your account automatically comes with the Danish Super Liga and Scottish Premier League plans at no cost.
Head over to the ‘Create an API Token’ section on your dashboard at MySportmonks to create one.
Once you have your API token, which is required for authentication and accessing the data endpoints, you must keep it private.
Chapter 2: Exploring top scorers
Using our Topscorers endpoint, you can retrieve accurate information about the goals, assists, and yellow cards. The endpoint returns the top 25 players for each category.
Top goal scorers can be retrieved using the season ID or stage ID. However, in today’s exercise, we shall apply only the former to retrieve top scorers in JSON response, which you can easily convert into other formats such as Excel.
Chapter 2.1 Retrieving Bundesliga Top Scorers
In the next section, we shall retrieve top scorers from the 2024/25 Bundesliga season using the season’s ID in conjunction with powerful inbuilt tools called include and filter.
You may go ahead and try this on your browser, using the API token that was created earlier. We will retrieve the same JSON response using Crystal.
require "http/client" require "json" API_URL = "https://api.sportmonks.com/v3/football/topscorers/seasons/23744" API_TOKEN = "API_Token" # Replace with your actual API token INCLUDE = "type;player:name,image_path;participant:name,image_path" FILTERS = "seasontopscorerTypes:208" # Construct the full URL url = "#{API_URL}?api_token=#{API_TOKEN}&include=#{INCLUDE}&filters=#{FILTERS}" # Make the HTTP GET request response = HTTP::Client.get(url) # Check if the response is successful if response.status_code == 200 parsed_response = JSON.parse(response.body) puts parsed_response.to_pretty_json else puts "Failed to fetch data: #{response.status_code}" end
{ "data": [ { "id": 165434073, "season_id": 23744, "player_id": 997, "type_id": 208, "position": 1, "total": 19, "participant_id": 503, "type": { "id": 208, "name": "Goal Topscorer", "code": "goal-topscorer", "developer_name": "GOAL_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 997, "country_id": 462, "sport_id": 1, "city_id": 51663, "position_id": 27, "detailed_position_id": 151, "nationality_id": 462, "name": "Harry Kane", "image_path": "https://cdn.sportmonks.com/images/soccer/players/5/997.png" }, "participant": { "id": 503, "sport_id": 1, "country_id": 11, "venue_id": 53, "name": "FC Bayern München", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/23/503.png" } }, { "id": 165055337, "season_id": 23744, "player_id": 294000, "type_id": 208, "position": 2, "total": 15, "participant_id": 366, "type": { "id": 208, "name": "Goal Topscorer", "code": "goal-topscorer", "developer_name": "GOAL_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 294000, "country_id": 886, "sport_id": 1, "city_id": null, "position_id": 27, "detailed_position_id": 151, "nationality_id": 886, "name": "Omar Marmoush", "image_path": "https://cdn.sportmonks.com/images/soccer/players/16/294000.png" }, "participant": { "id": 366, "sport_id": 1, "country_id": 11, "venue_id": 2169, "name": "Eintracht Frankfurt", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/14/366.png" } }, { "id": 178541021, "season_id": 23744, "player_id": 80675, "type_id": 208, "position": 3, "total": 14, "participant_id": 3321, "type": { "id": 208, "name": "Goal Topscorer", "code": "goal-topscorer", "developer_name": "GOAL_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 80675, "country_id": 245, "sport_id": 1, "city_id": null, "position_id": 27, "detailed_position_id": 151, "nationality_id": 245, "name": "Patrik Schick", "image_path": "https://cdn.sportmonks.com/images/soccer/players/3/80675.png" }, "participant": { "id": 3321, "sport_id": 1, "country_id": 11, "venue_id": 1947, "name": "Bayer 04 Leverkusen", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/25/3321.png" } }, { "id": 163825138, "season_id": 23744, "player_id": 34987, "type_id": 208, "position": 4, "total": 12, "participant_id": 683, "type": { "id": 208, "name": "Goal Topscorer", "code": "goal-topscorer", "developer_name": "GOAL_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 34987, "country_id": 11, "sport_id": 1, "city_id": null, "position_id": 27, "detailed_position_id": null, "nationality_id": 11, "name": "Tim Kleindienst", "image_path": "https://cdn.sportmonks.com/images/soccer/players/11/34987.png" }, "participant": { "id": 683, "sport_id": 1, "country_id": 11, "venue_id": 180, "name": "Borussia Mönchengladbach", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/11/683.png" } }, { "id": 165055349, "season_id": 23744, "player_id": 3862683, "type_id": 208, "position": 5, "total": 12, "participant_id": 794, "type": { "id": 208, "name": "Goal Topscorer", "code": "goal-topscorer", "developer_name": "GOAL_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 3862683, "country_id": 11, "sport_id": 1, "city_id": 22242, "position_id": 27, "detailed_position_id": null, "nationality_id": 11, "name": "Jonathan Burkardt", "image_path": "https://cdn.sportmonks.com/images/soccer/players/27/3862683.png" }, "participant": { "id": 794, "sport_id": 1, "country_id": 11, "venue_id": 2103, "name": "FSV Mainz 05", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/26/794.png" } }, { "id": 165055338, "season_id": 23744, "player_id": 37288979, "type_id": 208, "position": 6, "total": 11, "participant_id": 366, "type": { "id": 208, "name": "Goal Topscorer", "code": "goal-topscorer", "developer_name": "GOAL_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 37288979, "country_id": 17, "sport_id": 1, "city_id": 73757, "position_id": 27, "detailed_position_id": null, "nationality_id": 17, "name": "Hugo Ekitike", "image_path": "https://cdn.sportmonks.com/images/soccer/players/19/37288979.png" }, "participant": { "id": 366, "sport_id": 1, "country_id": 11, "venue_id": 2169, "name": "Eintracht Frankfurt", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/14/366.png" } }, { "id": 164309116, "season_id": 23744, "player_id": 33186829, "type_id": 208, "position": 7, "total": 10, "participant_id": 503, "type": { "id": 208, "name": "Goal Topscorer", "code": "goal-topscorer", "developer_name": "GOAL_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 33186829, "country_id": 11, "sport_id": 1, "city_id": 87094, "position_id": 26, "detailed_position_id": null, "nationality_id": 11, "name": "Jamal Musiala", "image_path": "https://cdn.sportmonks.com/images/soccer/players/13/33186829.png" }, "participant": { "id": 503, "sport_id": 1, "country_id": 11, "venue_id": 53, "name": "FC Bayern München", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/23/503.png" } }, { "id": 163825135, "season_id": 23744, "player_id": 37429246, "type_id": 208, "position": 8, "total": 9, "participant_id": 3321, "type": { "id": 208, "name": "Goal Topscorer", "code": "goal-topscorer", "developer_name": "GOAL_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 37429246, "country_id": 11, "sport_id": 1, "city_id": null, "position_id": 26, "detailed_position_id": 150, "nationality_id": 11, "name": "Florian Wirtz", "image_path": "https://cdn.sportmonks.com/images/soccer/players/30/37429246.png" }, "participant": { "id": 3321, "sport_id": 1, "country_id": 11, "venue_id": 1947, "name": "Bayer 04 Leverkusen", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/25/3321.png" } }, { "id": 169396640, "season_id": 23744, "player_id": 32695, "type_id": 208, "position": 9, "total": 9, "participant_id": 68, "type": { "id": 208, "name": "Goal Topscorer", "code": "goal-topscorer", "developer_name": "GOAL_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 32695, "country_id": 17, "sport_id": 1, "city_id": 3741, "position_id": 27, "detailed_position_id": 151, "nationality_id": 1703, "name": "Serhou Guirassy", "image_path": "https://cdn.sportmonks.com/images/soccer/players/23/32695.png" }, "participant": { "id": 68, "sport_id": 1, "country_id": 11, "venue_id": 2166, "name": "Borussia Dortmund", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/4/68.png" } }, { "id": 170324037, "season_id": 23744, "player_id": 37430769, "type_id": 208, "position": 10, "total": 8, "participant_id": 510, "type": { "id": 208, "name": "Goal Topscorer", "code": "goal-topscorer", "developer_name": "GOAL_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 37430769, "country_id": 614, "sport_id": 1, "city_id": null, "position_id": 27, "detailed_position_id": 151, "nationality_id": 614, "name": "Mohamed El Amine Amoura", "image_path": "https://cdn.sportmonks.com/images/soccer/placeholder.png" }, "participant": { "id": 510, "sport_id": 1, "country_id": 11, "venue_id": 80, "name": "VfL Wolfsburg", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/30/510.png" } }, { "id": 170324044, "season_id": 23744, "player_id": 537914, "type_id": 208, "position": 11, "total": 8, "participant_id": 510, "type": { "id": 208, "name": "Goal Topscorer", "code": "goal-topscorer", "developer_name": "GOAL_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 537914, "country_id": 320, "sport_id": 1, "city_id": null, "position_id": 27, "detailed_position_id": 151, "nationality_id": 320, "name": "Jonas Older Wind", "image_path": "https://cdn.sportmonks.com/images/soccer/players/26/537914.png" }, "participant": { "id": 510, "sport_id": 1, "country_id": 11, "venue_id": 80, "name": "VfL Wolfsburg", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/30/510.png" } }, { "id": 170324021, "season_id": 23744, "player_id": 24818280, "type_id": 208, "position": 12, "total": 8, "participant_id": 277, "type": { "id": 208, "name": "Goal Topscorer", "code": "goal-topscorer", "developer_name": "GOAL_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 24818280, "country_id": 1638, "sport_id": 1, "city_id": null, "position_id": 27, "detailed_position_id": 151, "nationality_id": 1638, "name": "Benjamin Šeško", "image_path": "https://cdn.sportmonks.com/images/soccer/players/8/24818280.png" }, "participant": { "id": 277, "sport_id": 1, "country_id": 11, "venue_id": 2171, "name": "RB Leipzig", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/21/277.png" } }, { "id": 163984991, "season_id": 23744, "player_id": 46092, "type_id": 208, "position": 13, "total": 8, "participant_id": 3319, "type": { "id": 208, "name": "Goal Topscorer", "code": "goal-topscorer", "developer_name": "GOAL_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 46092, "country_id": 11, "sport_id": 1, "city_id": 35451, "position_id": 27, "detailed_position_id": 151, "nationality_id": 507, "name": "Ermedin Demirović", "image_path": "https://cdn.sportmonks.com/images/soccer/players/12/46092.png" }, "participant": { "id": 3319, "sport_id": 1, "country_id": 11, "venue_id": 1945, "name": "VfB Stuttgart", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/23/3319.png" } }, { "id": 170887116, "season_id": 23744, "player_id": 84658, "type_id": 208, "position": 14, "total": 7, "participant_id": 82, "type": { "id": 208, "name": "Goal Topscorer", "code": "goal-topscorer", "developer_name": "GOAL_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 84658, "country_id": 320, "sport_id": 1, "city_id": null, "position_id": 26, "detailed_position_id": null, "nationality_id": 320, "name": "Jens Stage", "image_path": "https://cdn.sportmonks.com/images/soccer/players/18/84658.png" }, "participant": { "id": 82, "sport_id": 1, "country_id": 11, "venue_id": 2172, "name": "Werder Bremen", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/18/82.png" } }, { "id": 164081514, "season_id": 23744, "player_id": 37316840, "type_id": 208, "position": 15, "total": 7, "participant_id": 68, "type": { "id": 208, "name": "Goal Topscorer", "code": "goal-topscorer", "developer_name": "GOAL_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 37316840, "country_id": 462, "sport_id": 1, "city_id": null, "position_id": 27, "detailed_position_id": 152, "nationality_id": 462, "name": "Jamie Bynoe-Gittens", "image_path": "https://cdn.sportmonks.com/images/soccer/players/8/37316840.png" }, "participant": { "id": 68, "sport_id": 1, "country_id": 11, "venue_id": 2166, "name": "Borussia Dortmund", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/4/68.png" } }, { "id": 163984985, "season_id": 23744, "player_id": 1232, "type_id": 208, "position": 16, "total": 7, "participant_id": 2726, "type": { "id": 208, "name": "Goal Topscorer", "code": "goal-topscorer", "developer_name": "GOAL_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 1232, "country_id": 266, "sport_id": 1, "city_id": 101419, "position_id": 27, "detailed_position_id": 151, "nationality_id": 266, "name": "Andrej Kramarić", "image_path": "https://cdn.sportmonks.com/images/soccer/players/16/1232.png" }, "participant": { "id": 2726, "sport_id": 1, "country_id": 11, "venue_id": 1618, "name": "TSG Hoffenheim", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/6/2726.png" } }, { "id": 163984995, "season_id": 23744, "player_id": 10959317, "type_id": 208, "position": 17, "total": 7, "participant_id": 3611, "type": { "id": 208, "name": "Goal Topscorer", "code": "goal-topscorer", "developer_name": "GOAL_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 10959317, "country_id": 479, "sport_id": 1, "city_id": 39685, "position_id": 27, "detailed_position_id": 151, "nationality_id": 479, "name": "Shuto Machino", "image_path": "https://cdn.sportmonks.com/images/soccer/players/21/10959317.png" }, "participant": { "id": 3611, "sport_id": 1, "country_id": 11, "venue_id": 2115, "name": "Holstein Kiel", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/27/3611.png" } }, { "id": 167635137, "season_id": 23744, "player_id": 31991374, "type_id": 208, "position": 18, "total": 7, "participant_id": 3321, "type": { "id": 208, "name": "Goal Topscorer", "code": "goal-topscorer", "developer_name": "GOAL_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 31991374, "country_id": 716, "sport_id": 1, "city_id": null, "position_id": 27, "detailed_position_id": 151, "nationality_id": 716, "name": "Victor Okoh Boniface", "image_path": "https://cdn.sportmonks.com/images/soccer/players/14/31991374.png" }, "participant": { "id": 3321, "sport_id": 1, "country_id": 11, "venue_id": 1947, "name": "Bayer 04 Leverkusen", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/25/3321.png" } }, { "id": 167635168, "season_id": 23744, "player_id": 41077, "type_id": 208, "position": 19, "total": 7, "participant_id": 3319, "type": { "id": 208, "name": "Goal Topscorer", "code": "goal-topscorer", "developer_name": "GOAL_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 41077, "country_id": 11, "sport_id": 1, "city_id": null, "position_id": 27, "detailed_position_id": null, "nationality_id": 11, "name": "Deniz Undav", "image_path": "https://cdn.sportmonks.com/images/soccer/players/21/41077.png" }, "participant": { "id": 3319, "sport_id": 1, "country_id": 11, "venue_id": 1945, "name": "VfB Stuttgart", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/23/3319.png" } }, { "id": 178541017, "season_id": 23744, "player_id": 37266227, "type_id": 208, "position": 20, "total": 7, "participant_id": 3611, "type": { "id": 208, "name": "Goal Topscorer", "code": "goal-topscorer", "developer_name": "GOAL_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 37266227, "country_id": 11, "sport_id": 1, "city_id": null, "position_id": 27, "detailed_position_id": null, "nationality_id": 11, "name": "Phil Harres", "image_path": "https://cdn.sportmonks.com/images/soccer/placeholder.png" }, "participant": { "id": 3611, "sport_id": 1, "country_id": 11, "venue_id": 2115, "name": "Holstein Kiel", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/27/3611.png" } }, { "id": 167759761, "season_id": 23744, "player_id": 24799984, "type_id": 208, "position": 21, "total": 6, "participant_id": 503, "type": { "id": 208, "name": "Goal Topscorer", "code": "goal-topscorer", "developer_name": "GOAL_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 24799984, "country_id": 462, "sport_id": 1, "city_id": null, "position_id": 26, "detailed_position_id": null, "nationality_id": 17, "name": "Michael Olise", "image_path": "https://cdn.sportmonks.com/images/soccer/players/16/24799984.png" }, "participant": { "id": 503, "sport_id": 1, "country_id": 11, "venue_id": 53, "name": "FC Bayern München", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/23/503.png" } }, { "id": 165172754, "season_id": 23744, "player_id": 539993, "type_id": 208, "position": 22, "total": 6, "participant_id": 277, "type": { "id": 208, "name": "Goal Topscorer", "code": "goal-topscorer", "developer_name": "GOAL_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 539993, "country_id": 556, "sport_id": 1, "city_id": null, "position_id": 27, "detailed_position_id": 151, "nationality_id": 556, "name": "Lois Openda", "image_path": "https://cdn.sportmonks.com/images/soccer/players/25/539993.png" }, "participant": { "id": 277, "sport_id": 1, "country_id": 11, "venue_id": 2171, "name": "RB Leipzig", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/21/277.png" } }, { "id": 168074185, "season_id": 23744, "player_id": 31757, "type_id": 208, "position": 23, "total": 6, "participant_id": 82, "type": { "id": 208, "name": "Goal Topscorer", "code": "goal-topscorer", "developer_name": "GOAL_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 31757, "country_id": 11, "sport_id": 1, "city_id": 24229, "position_id": 27, "detailed_position_id": 151, "nationality_id": 11, "name": "Marvin Ducksch", "image_path": "https://cdn.sportmonks.com/images/soccer/players/13/31757.png" }, "participant": { "id": 82, "sport_id": 1, "country_id": 11, "venue_id": 2172, "name": "Werder Bremen", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/18/82.png" } }, { "id": 163984992, "season_id": 23744, "player_id": 310486, "type_id": 208, "position": 24, "total": 6, "participant_id": 3543, "type": { "id": 208, "name": "Goal Topscorer", "code": "goal-topscorer", "developer_name": "GOAL_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 310486, "country_id": 479, "sport_id": 1, "city_id": 2349, "position_id": 27, "detailed_position_id": 156, "nationality_id": 479, "name": "Ritsu Doan", "image_path": "https://cdn.sportmonks.com/images/soccer/players/22/310486.png" }, "participant": { "id": 3543, "sport_id": 1, "country_id": 11, "venue_id": 2063, "name": "SC Freiburg", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/23/3543.png" } }, { "id": 167635169, "season_id": 23744, "player_id": 95844, "type_id": 208, "position": 25, "total": 6, "participant_id": 683, "type": { "id": 208, "name": "Goal Topscorer", "code": "goal-topscorer", "developer_name": "GOAL_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 95844, "country_id": 17, "sport_id": 1, "city_id": 50419, "position_id": 27, "detailed_position_id": 151, "nationality_id": 17, "name": "Alassane Pléa", "image_path": "https://cdn.sportmonks.com/images/soccer/players/4/95844.png" }, "participant": { "id": 683, "sport_id": 1, "country_id": 11, "venue_id": 180, "name": "Borussia Mönchengladbach", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/11/683.png" } }
Explanation
This program performs a setup of the API details by defining the base API URL and query parameters. It then constructs the full API URL before sending an HTTP GET Request to the specified endpoint.
The response is checked, and if the server responds with a 200-status code, the response body is parsed as JSON. The parsed JSON is then printed in a pretty, human-readable format using the to_pretty_json method.
Using the filter statement seasontopscorerTypes with the value of 208 returns the highest goal scorer for the 2024/25 Bundesliga season.
As you can see from the self-descriptive field in the response, among them are the player’s ID, total, and type ID. A full description of the response can be found on our documentation page.
Chapter 2.2: Formatting our response to HTML
We shall format our response for improved readability using HTML by making a few changes to the piece of code from 2.1.
Using the same URL, the modified code is provided below.
As you can see, upon success, the JSON data is parsed, and key details such as player name, total assists, team name, and player image are rendered into a div tag in HTML.
require "http/client" require "json" API_URL = "https://api.sportmonks.com/v3/football/topscorers/seasons/23744" API_TOKEN = "API Token" # Replace with your actual API token INCLUDE = "type;player:name,image_path;participant:name,image_path" FILTERS = "seasontopscorerTypes:208" # Construct the full URL url = "#{API_URL}?api_token=#{API_TOKEN}&include=#{INCLUDE}&filters=#{FILTERS}" # Make the HTTP GET request response = HTTP::Client.get(url) # Check if the response is successful if response.status_code == 200 parsed_response = JSON.parse(response.body) parsed_response["data"].as_a.each do |entry| player_name = entry["player"]["name"].as_s total_goals = entry["total"].as_i team_name = entry["participant"]["name"].as_s image_path = entry["player"]["image_path"].as_s puts <<-HTML <div> <img decoding="async" src="#{image_path}" width="50" height="50" alt="Player Image"> <p>Name: #{player_name}</p> <p>Goals: #{total_goals}</p> <p>Team: #{team_name}</p> <br><br> </div> HTML end else puts "Failed to fetch data: #{response.status_code}" end
Chapter 3 Exploring Assists in Bundesliga
Assists are just as important as goals themselves, and with Sportmonks Football API, retrieving assists is as easy as ABC. Using the type ID 209, we can easily find out who the top assist makers are in the Bundesliga.
Chapter 3.1 Retrieving Top Assists
Using a modified version of the code from Chapter 2.1 and the URL below, we shall retrieve another JSON response showing top assists for the 2024/25 Bundesliga season.
require "http/client" require "json" API_URL = "https://api.sportmonks.com/v3/football/topscorers/seasons/23744" API_TOKEN = "API_Token" # Replace with your actual API token INCLUDE = "type;player:name,image_path;participant:name,image_path" FILTERS = "seasontopscorerTypes:209" # Construct the full URL url = "#{API_URL}?api_token=#{API_TOKEN}&include=#{INCLUDE}&filters=#{FILTERS}" # Make the HTTP GET request response = HTTP::Client.get(url) # Check if the response is successful if response.status_code == 200 parsed_response = JSON.parse(response.body) puts parsed_response.to_pretty_json else puts "Failed to fetch data: #{response.status_code}" end
{ "data": [ { "id": 165172726, "season_id": 23744, "player_id": 37429246, "type_id": 209, "position": 1, "total": 10, "participant_id": 3321, "type": { "id": 209, "name": "Assist Topscorer", "code": "assist-topscorer", "developer_name": "ASSIST_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 37429246, "country_id": 11, "sport_id": 1, "city_id": null, "position_id": 26, "detailed_position_id": 150, "nationality_id": 11, "name": "Florian Wirtz", "image_path": "https://cdn.sportmonks.com/images/soccer/players/30/37429246.png" }, "participant": { "id": 3321, "sport_id": 1, "country_id": 11, "venue_id": 1947, "name": "Bayer 04 Leverkusen", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/25/3321.png" } }, { "id": 165055315, "season_id": 23744, "player_id": 294000, "type_id": 209, "position": 2, "total": 9, "participant_id": 366, "type": { "id": 209, "name": "Assist Topscorer", "code": "assist-topscorer", "developer_name": "ASSIST_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 294000, "country_id": 886, "sport_id": 1, "city_id": null, "position_id": 27, "detailed_position_id": 151, "nationality_id": 886, "name": "Omar Marmoush", "image_path": "https://cdn.sportmonks.com/images/soccer/players/16/294000.png" }, "participant": { "id": 366, "sport_id": 1, "country_id": 11, "venue_id": 2169, "name": "Eintracht Frankfurt", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/14/366.png" } }, { "id": 168844779, "season_id": 23744, "player_id": 24799984, "type_id": 209, "position": 3, "total": 8, "participant_id": 503, "type": { "id": 209, "name": "Assist Topscorer", "code": "assist-topscorer", "developer_name": "ASSIST_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 24799984, "country_id": 462, "sport_id": 1, "city_id": null, "position_id": 26, "detailed_position_id": null, "nationality_id": 17, "name": "Michael Olise", "image_path": "https://cdn.sportmonks.com/images/soccer/players/16/24799984.png" }, "participant": { "id": 503, "sport_id": 1, "country_id": 11, "venue_id": 53, "name": "FC Bayern München", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/23/503.png" } }, { "id": 163984975, "season_id": 23744, "player_id": 31913, "type_id": 209, "position": 4, "total": 8, "participant_id": 3543, "type": { "id": 209, "name": "Assist Topscorer", "code": "assist-topscorer", "developer_name": "ASSIST_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 31913, "country_id": 11, "sport_id": 1, "city_id": 68636, "position_id": 27, "detailed_position_id": 152, "nationality_id": 251, "name": "Vincenzo Grifo", "image_path": "https://cdn.sportmonks.com/images/soccer/players/9/31913.png" }, "participant": { "id": 3543, "sport_id": 1, "country_id": 11, "venue_id": 2063, "name": "SC Freiburg", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/23/3543.png" } }, { "id": 164309107, "season_id": 23744, "player_id": 997, "type_id": 209, "position": 5, "total": 7, "participant_id": 503, "type": { "id": 209, "name": "Assist Topscorer", "code": "assist-topscorer", "developer_name": "ASSIST_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 997, "country_id": 462, "sport_id": 1, "city_id": 51663, "position_id": 27, "detailed_position_id": 151, "nationality_id": 462, "name": "Harry Kane", "image_path": "https://cdn.sportmonks.com/images/soccer/players/5/997.png" }, "participant": { "id": 503, "sport_id": 1, "country_id": 11, "venue_id": 53, "name": "FC Bayern München", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/23/503.png" } }, { "id": 170323959, "season_id": 23744, "player_id": 539993, "type_id": 209, "position": 6, "total": 7, "participant_id": 277, "type": { "id": 209, "name": "Assist Topscorer", "code": "assist-topscorer", "developer_name": "ASSIST_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 539993, "country_id": 556, "sport_id": 1, "city_id": null, "position_id": 27, "detailed_position_id": 151, "nationality_id": 556, "name": "Lois Openda", "image_path": "https://cdn.sportmonks.com/images/soccer/players/25/539993.png" }, "participant": { "id": 277, "sport_id": 1, "country_id": 11, "venue_id": 2171, "name": "RB Leipzig", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/21/277.png" } }, { "id": 170886997, "season_id": 23744, "player_id": 31757, "type_id": 209, "position": 7, "total": 7, "participant_id": 82, "type": { "id": 209, "name": "Assist Topscorer", "code": "assist-topscorer", "developer_name": "ASSIST_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 31757, "country_id": 11, "sport_id": 1, "city_id": 24229, "position_id": 27, "detailed_position_id": 151, "nationality_id": 11, "name": "Marvin Ducksch", "image_path": "https://cdn.sportmonks.com/images/soccer/players/13/31757.png" }, "participant": { "id": 82, "sport_id": 1, "country_id": 11, "venue_id": 2172, "name": "Werder Bremen", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/18/82.png" } }, { "id": 167635130, "season_id": 23744, "player_id": 1887, "type_id": 209, "position": 8, "total": 6, "participant_id": 3321, "type": { "id": 209, "name": "Assist Topscorer", "code": "assist-topscorer", "developer_name": "ASSIST_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 1887, "country_id": 62, "sport_id": 1, "city_id": 6977, "position_id": 26, "detailed_position_id": 149, "nationality_id": 62, "name": "Granit Xhaka", "image_path": "https://cdn.sportmonks.com/images/soccer/players/31/1887.png" }, "participant": { "id": 3321, "sport_id": 1, "country_id": 11, "venue_id": 1947, "name": "Bayer 04 Leverkusen", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/25/3321.png" } }, { "id": 174391865, "season_id": 23744, "player_id": 32362, "type_id": 209, "position": 9, "total": 6, "participant_id": 503, "type": { "id": 209, "name": "Assist Topscorer", "code": "assist-topscorer", "developer_name": "ASSIST_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 32362, "country_id": 11, "sport_id": 1, "city_id": 75833, "position_id": 26, "detailed_position_id": 149, "nationality_id": 11, "name": "Joshua Kimmich", "image_path": "https://cdn.sportmonks.com/images/soccer/players/10/32362.png" }, "participant": { "id": 503, "sport_id": 1, "country_id": 11, "venue_id": 53, "name": "FC Bayern München", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/23/503.png" } }, { "id": 163984976, "season_id": 23744, "player_id": 31636, "type_id": 209, "position": 10, "total": 6, "participant_id": 82, "type": { "id": 209, "name": "Assist Topscorer", "code": "assist-topscorer", "developer_name": "ASSIST_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 31636, "country_id": 11, "sport_id": 1, "city_id": 91802, "position_id": 25, "detailed_position_id": 154, "nationality_id": 11, "name": "Mitchell Weiser", "image_path": "https://cdn.sportmonks.com/images/soccer/players/20/31636.png" }, "participant": { "id": 82, "sport_id": 1, "country_id": 11, "venue_id": 2172, "name": "Werder Bremen", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/18/82.png" } }, { "id": 167635124, "season_id": 23744, "player_id": 37430769, "type_id": 209, "position": 11, "total": 6, "participant_id": 510, "type": { "id": 209, "name": "Assist Topscorer", "code": "assist-topscorer", "developer_name": "ASSIST_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 37430769, "country_id": 614, "sport_id": 1, "city_id": null, "position_id": 27, "detailed_position_id": 151, "nationality_id": 614, "name": "Mohamed El Amine Amoura", "image_path": "https://cdn.sportmonks.com/images/soccer/placeholder.png" }, "participant": { "id": 510, "sport_id": 1, "country_id": 11, "venue_id": 80, "name": "VfL Wolfsburg", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/30/510.png" } }, { "id": 168655624, "season_id": 23744, "player_id": 35141, "type_id": 209, "position": 12, "total": 5, "participant_id": 794, "type": { "id": 209, "name": "Assist Topscorer", "code": "assist-topscorer", "developer_name": "ASSIST_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 35141, "country_id": 143, "sport_id": 1, "city_id": null, "position_id": 25, "detailed_position_id": 155, "nationality_id": 143, "name": "Philipp Mwene", "image_path": "https://cdn.sportmonks.com/images/soccer/players/5/35141.png" }, "participant": { "id": 794, "sport_id": 1, "country_id": 11, "venue_id": 2103, "name": "FSV Mainz 05", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/26/794.png" } }, { "id": 169014761, "season_id": 23744, "player_id": 37266013, "type_id": 209, "position": 13, "total": 5, "participant_id": 366, "type": { "id": 209, "name": "Assist Topscorer", "code": "assist-topscorer", "developer_name": "ASSIST_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 37266013, "country_id": 11, "sport_id": 1, "city_id": null, "position_id": 27, "detailed_position_id": null, "nationality_id": 11, "name": "Ansgar Knauff", "image_path": "https://cdn.sportmonks.com/images/soccer/players/29/37266013.png" }, "participant": { "id": 366, "sport_id": 1, "country_id": 11, "venue_id": 2169, "name": "Eintracht Frankfurt", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/14/366.png" } }, { "id": 163984979, "season_id": 23744, "player_id": 160208, "type_id": 209, "position": 14, "total": 5, "participant_id": 3321, "type": { "id": 209, "name": "Assist Topscorer", "code": "assist-topscorer", "developer_name": "ASSIST_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 160208, "country_id": 32, "sport_id": 1, "city_id": 93634, "position_id": 25, "detailed_position_id": 155, "nationality_id": 32, "name": "Alejandro Grimaldo García", "image_path": "https://cdn.sportmonks.com/images/soccer/players/16/160208.png" }, "participant": { "id": 3321, "sport_id": 1, "country_id": 11, "venue_id": 1947, "name": "Bayer 04 Leverkusen", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/25/3321.png" } }, { "id": 169396550, "season_id": 23744, "player_id": 335673, "type_id": 209, "position": 15, "total": 5, "participant_id": 3321, "type": { "id": 209, "name": "Assist Topscorer", "code": "assist-topscorer", "developer_name": "ASSIST_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 335673, "country_id": 44, "sport_id": 1, "city_id": null, "position_id": 26, "detailed_position_id": 153, "nationality_id": 44, "name": "Exequiel Alejandro Palacios", "image_path": "https://cdn.sportmonks.com/images/soccer/players/25/335673.png" }, "participant": { "id": 3321, "sport_id": 1, "country_id": 11, "venue_id": 1947, "name": "Bayer 04 Leverkusen", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/25/3321.png" } }, { "id": 165055307, "season_id": 23744, "player_id": 34987, "type_id": 209, "position": 16, "total": 5, "participant_id": 683, "type": { "id": 209, "name": "Assist Topscorer", "code": "assist-topscorer", "developer_name": "ASSIST_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 34987, "country_id": 11, "sport_id": 1, "city_id": null, "position_id": 27, "detailed_position_id": null, "nationality_id": 11, "name": "Tim Kleindienst", "image_path": "https://cdn.sportmonks.com/images/soccer/players/11/34987.png" }, "participant": { "id": 683, "sport_id": 1, "country_id": 11, "venue_id": 180, "name": "Borussia Mönchengladbach", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/11/683.png" } }, { "id": 167440062, "season_id": 23744, "player_id": 32158, "type_id": 209, "position": 17, "total": 5, "participant_id": 68, "type": { "id": 209, "name": "Assist Topscorer", "code": "assist-topscorer", "developer_name": "ASSIST_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 32158, "country_id": 11, "sport_id": 1, "city_id": 11918, "position_id": 26, "detailed_position_id": null, "nationality_id": 11, "name": "Julian Brandt", "image_path": "https://cdn.sportmonks.com/images/soccer/players/30/32158.png" }, "participant": { "id": 68, "sport_id": 1, "country_id": 11, "venue_id": 2166, "name": "Borussia Dortmund", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/4/68.png" } }, { "id": 170323982, "season_id": 23744, "player_id": 6605, "type_id": 209, "position": 18, "total": 5, "participant_id": 353, "type": { "id": 209, "name": "Assist Topscorer", "code": "assist-topscorer", "developer_name": "ASSIST_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 6605, "country_id": 98, "sport_id": 1, "city_id": 56136, "position_id": 26, "detailed_position_id": 153, "nationality_id": 98, "name": "Jackson Irvine", "image_path": "https://cdn.sportmonks.com/images/soccer/players/13/6605.png" }, "participant": { "id": 353, "sport_id": 1, "country_id": 11, "venue_id": 2110, "name": "St. Pauli", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/1/353.png" } }, { "id": 164309101, "season_id": 23744, "player_id": 62502, "type_id": 209, "position": 19, "total": 5, "participant_id": 68, "type": { "id": 209, "name": "Assist Topscorer", "code": "assist-topscorer", "developer_name": "ASSIST_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 62502, "country_id": 614, "sport_id": 1, "city_id": 19955, "position_id": 25, "detailed_position_id": 155, "nationality_id": 614, "name": "Ramy Bensebaini", "image_path": "https://cdn.sportmonks.com/images/soccer/players/6/62502.png" }, "participant": { "id": 68, "sport_id": 1, "country_id": 11, "venue_id": 2166, "name": "Borussia Dortmund", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/4/68.png" } }, { "id": 179127397, "season_id": 23744, "player_id": 4536572, "type_id": 209, "position": 20, "total": 4, "participant_id": 3319, "type": { "id": 209, "name": "Assist Topscorer", "code": "assist-topscorer", "developer_name": "ASSIST_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 4536572, "country_id": 11, "sport_id": 1, "city_id": null, "position_id": 26, "detailed_position_id": 149, "nationality_id": 11, "name": "Angelo Stiller", "image_path": "https://cdn.sportmonks.com/images/soccer/players/28/4536572.png" }, "participant": { "id": 3319, "sport_id": 1, "country_id": 11, "venue_id": 1945, "name": "VfB Stuttgart", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/23/3319.png" } }, { "id": 177132532, "season_id": 23744, "player_id": 84604, "type_id": 209, "position": 21, "total": 4, "participant_id": 510, "type": { "id": 209, "name": "Assist Topscorer", "code": "assist-topscorer", "developer_name": "ASSIST_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 84604, "country_id": 320, "sport_id": 1, "city_id": null, "position_id": 25, "detailed_position_id": 155, "nationality_id": 320, "name": "Joakim Mæhle", "image_path": "https://cdn.sportmonks.com/images/soccer/players/28/84604.png" }, "participant": { "id": 510, "sport_id": 1, "country_id": 11, "venue_id": 80, "name": "VfL Wolfsburg", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/30/510.png" } }, { "id": 164309106, "season_id": 23744, "player_id": 37259654, "type_id": 209, "position": 22, "total": 4, "participant_id": 510, "type": { "id": 209, "name": "Assist Topscorer", "code": "assist-topscorer", "developer_name": "ASSIST_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 37259654, "country_id": 143, "sport_id": 1, "city_id": 92154, "position_id": 26, "detailed_position_id": 156, "nationality_id": 143, "name": "Patrick Wimmer", "image_path": "https://cdn.sportmonks.com/images/soccer/players/6/37259654.png" }, "participant": { "id": 510, "sport_id": 1, "country_id": 11, "venue_id": 80, "name": "VfL Wolfsburg", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/30/510.png" } }, { "id": 170323998, "season_id": 23744, "player_id": 38673, "type_id": 209, "position": 23, "total": 4, "participant_id": 353, "type": { "id": 209, "name": "Assist Topscorer", "code": "assist-topscorer", "developer_name": "ASSIST_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 38673, "country_id": 11, "sport_id": 1, "city_id": null, "position_id": 27, "detailed_position_id": 151, "nationality_id": 11, "name": "Johannes Eggestein", "image_path": "https://cdn.sportmonks.com/images/soccer/players/17/38673.png" }, "participant": { "id": 353, "sport_id": 1, "country_id": 11, "venue_id": 2110, "name": "St. Pauli", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/1/353.png" } }, { "id": 184553400, "season_id": 23744, "player_id": 37538035, "type_id": 209, "position": 24, "total": 4, "participant_id": 366, "type": { "id": 209, "name": "Assist Topscorer", "code": "assist-topscorer", "developer_name": "ASSIST_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 37538035, "country_id": 11, "sport_id": 1, "city_id": null, "position_id": 25, "detailed_position_id": null, "nationality_id": 11, "name": "Nathaniel Brown", "image_path": "https://cdn.sportmonks.com/images/soccer/players/19/37538035.png" }, "participant": { "id": 366, "sport_id": 1, "country_id": 11, "venue_id": 2169, "name": "Eintracht Frankfurt", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/14/366.png" } }, { "id": 174205231, "season_id": 23744, "player_id": 310486, "type_id": 209, "position": 25, "total": 4, "participant_id": 3543, "type": { "id": 209, "name": "Assist Topscorer", "code": "assist-topscorer", "developer_name": "ASSIST_TOPSCORER", "model_type": "statistic", "stat_group": null }, "player": { "id": 310486, "country_id": 479, "sport_id": 1, "city_id": 2349, "position_id": 27, "detailed_position_id": 156, "nationality_id": 479, "name": "Ritsu Doan", "image_path": "https://cdn.sportmonks.com/images/soccer/players/22/310486.png" }, "participant": { "id": 3543, "sport_id": 1, "country_id": 11, "venue_id": 2063, "name": "SC Freiburg", "image_path": "https://cdn.sportmonks.com/images/soccer/teams/23/3543.png" } } ], "pagination": { "count": 25, "per_page": 25, "current_page": 1, "next_page": "https://api.sportmonks.com/v3/football/topscorers/seasons/23744?include=type%3Bplayer%3Aname%2Cimage_path%3Bparticipant%3Aname%2Cimage_path&filters=seasontopscorerTypes%3A209&page=2", "has_more": true }, "subscription": [ { "meta": { "trial_ends_at": "2024-10-16 14:50:45", "ends_at": null, "current_timestamp": 1738953405 }, "plans": [ { "plan": "Enterprise plan (loyal)", "sport": "Football", "category": "Advanced" }, { "plan": "Enterprise Plan", "sport": "Cricket", "category": "Standard" }, { "plan": "Formula One", "sport": "Formula One", "category": "Standard" } ], "add_ons": [ { "add_on": "All-in News API", "sport": "Football", "category": "News" }, { "add_on": "pressure index add-on", "sport": "Football", "category": "Default" }, { "add_on": "Enterprise Plan Predictions", "sport": "Football", "category": "Predictions" }, { "add_on": "xG Advanced", "sport": "Football", "category": "Expected" } ], "widgets": [ { "widget": "Sportmonks Widgets", "sport": "Football" } ] } ], "rate_limit": { "resets_in_seconds": 2228, "remaining": 2995, "requested_entity": "Topscorer" }, "timezone": "UTC" }
Chapter 3.2 Formatting Our Response to HTML
As we did in Chapter 2.2, we shall format our response for improved readability using HTML by making slight adjustments.
Using the same URL from section 3.1, the modified code is provided below.
require "http/client" require "json" API_URL = "https://api.sportmonks.com/v3/football/topscorers/seasons/23744" API_TOKEN = "API Token" # Replace with your actual API token INCLUDE = "type;player:name,image_path;participant:name,image_path" FILTERS = "seasontopscorerTypes:209" # Construct the full URL url = "#{API_URL}?api_token=#{API_TOKEN}&include=#{INCLUDE}&filters=#{FILTERS}" # Make the HTTP GET request response = HTTP::Client.get(url) # Check if the response is successful if response.status_code == 200 parsed_response = JSON.parse(response.body) parsed_response["data"].as_a.each do |entry| player_name = entry["player"]["name"].as_s total_assists = entry["total"].as_i # This should now represent assists team_name = entry["participant"]["name"].as_s image_path = entry["player"]["image_path"].as_s
As you can see, upon success, the JSON data is parsed, and key details like the player name, total assists, team name, and player image are rendered into a div tag in HTML. If the API request fails, an error message is displayed.
Unleash league-leading football data with Sportmonks
As with so many other deep football-related statistics, retrieving top scorers from any league of interest from our wide coverage is a breeze with Sportmonks’ Football API.
In the few chapters we covered, we could do this easily with the Topscorers’ endpoint. We also demonstrated how to retrieve associated player names for both top scorers and assists in the Bundesliga using Crystal, one of the many programming languages supported by our Football API.
As you can see, Sportmonks offers an incredible treasure trove of deep football-related statistics via its API, which is continuously improved and updated to provide exceptional value for money.
What are you waiting for? Why not sign up for a free trial today?
FAQ
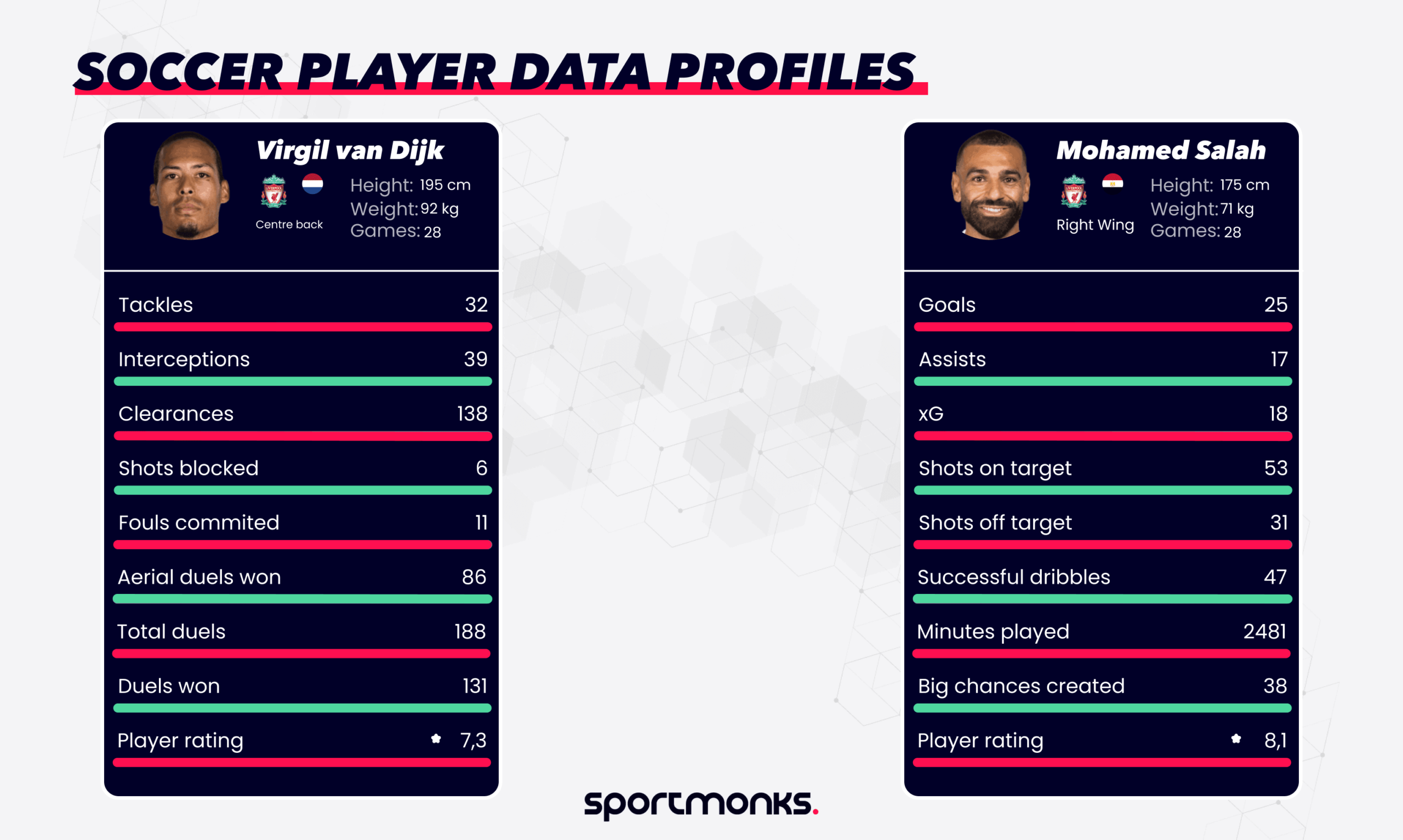
How to Build soccer player data profiles using Sportmonks Football API
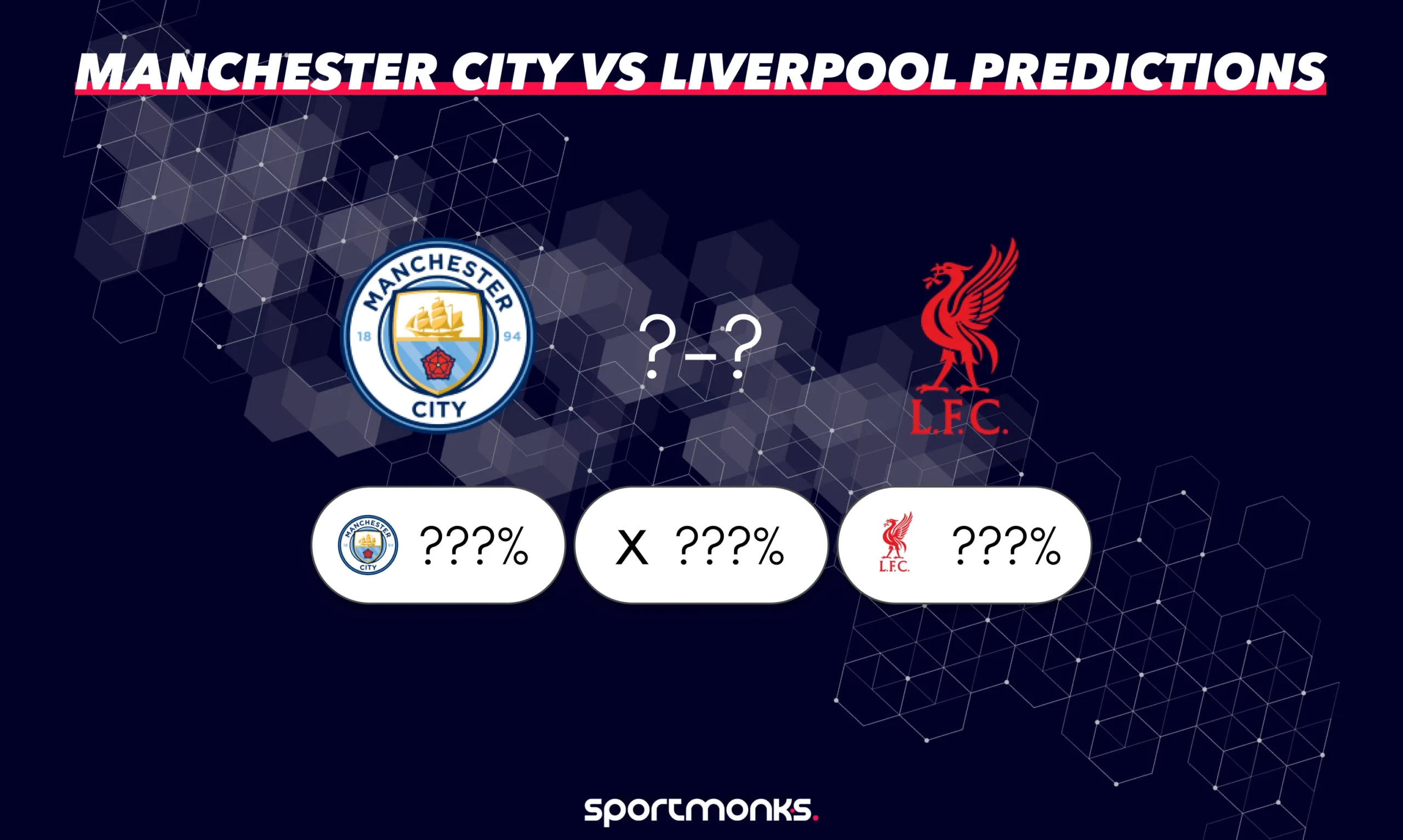
Manchester City vs Liverpool Predictions with Sportmonks data
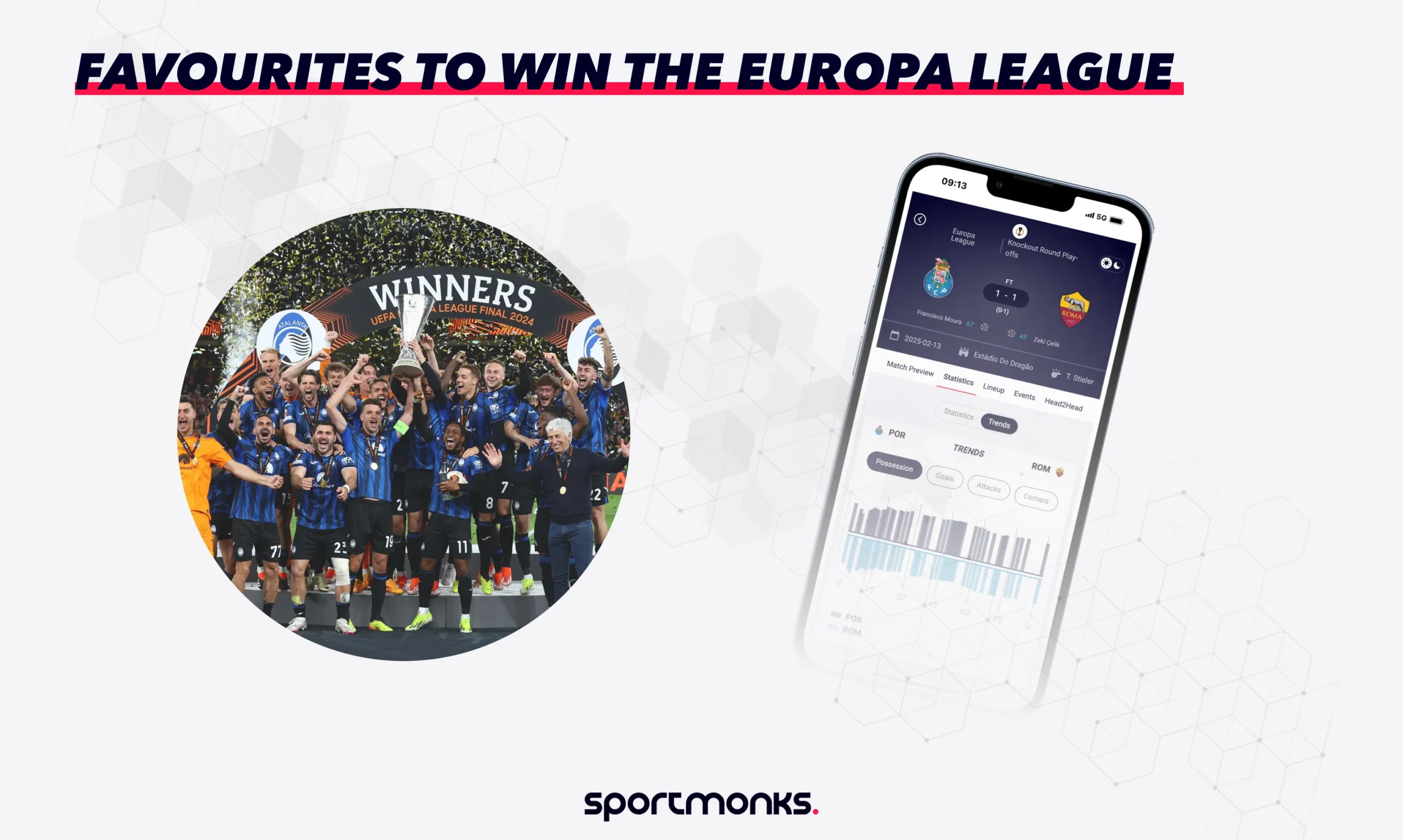
Who is the favourite to become Europa League champions based on Sportmonks data?
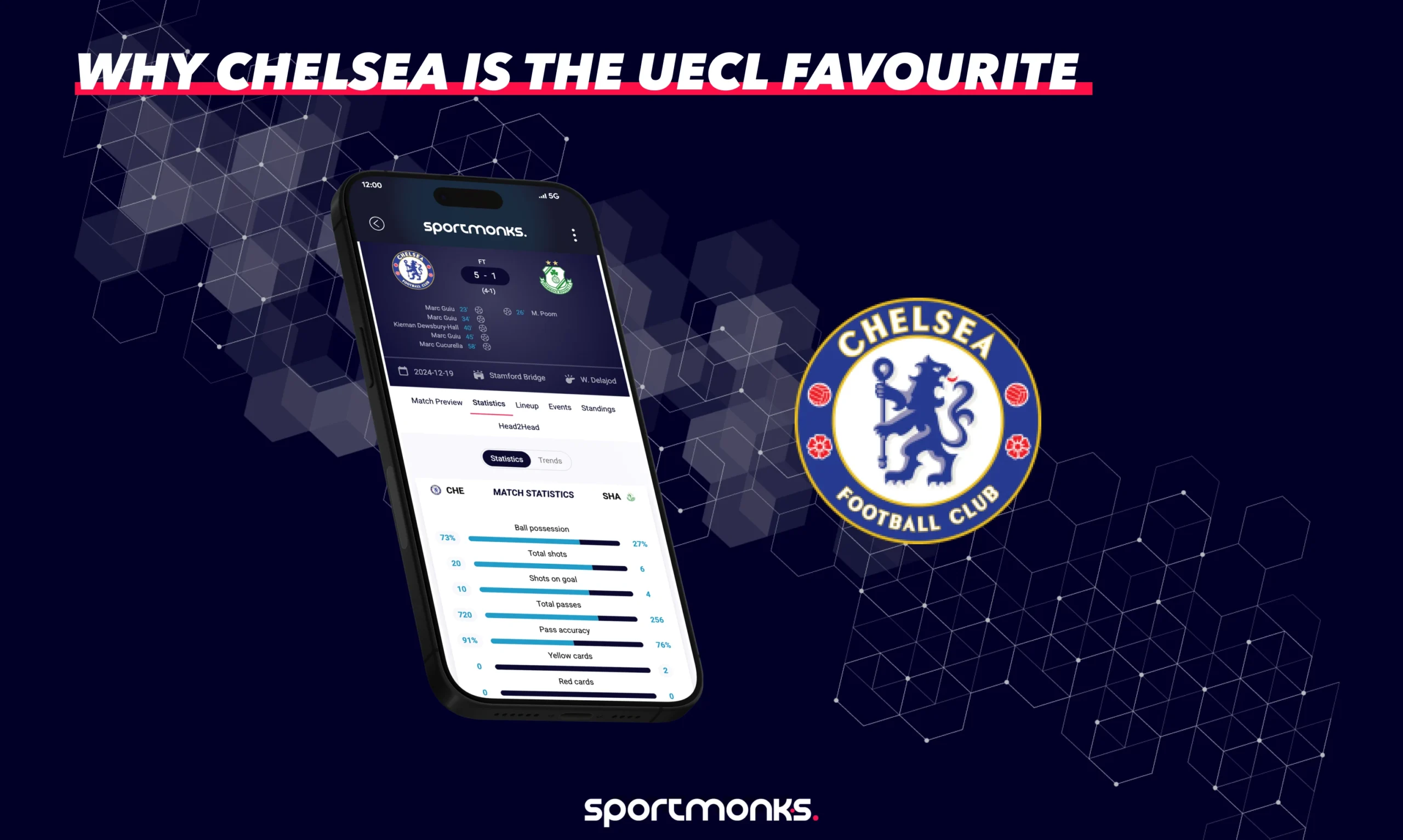
Europa Conference League Predictions: 5 Reasons Chelsea is the top favourite
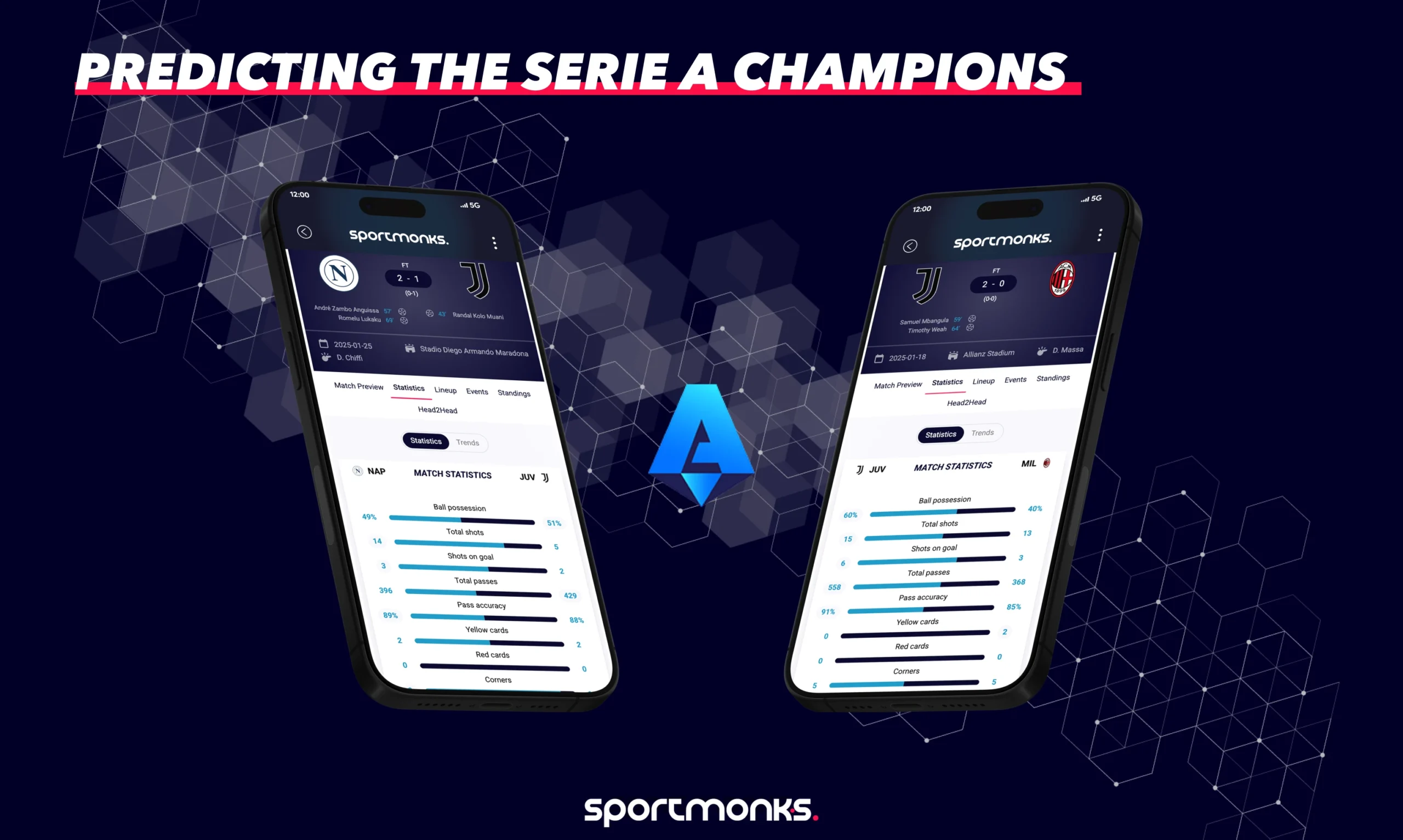